Image processing
Contents
Our task is, to find the red whiteboard markers on the following photo.
clear all imag1=imread('047.jpg');
This is a 3 dimensional array. It contains for each pixel the intensity of the colors in RGB order (red, green, blue)
imag1(1,1,:)
1×1×3 uint8 array ans(:,:,1) = 182 ans(:,:,2) = 169 ans(:,:,3) = 135
The size of the image for the first two dimension is the number of pixels
size(imag1)
ans = 3456 4608 3
It contains unassigned integers to save space
whos
Name Size Bytes Class Attributes ans 1x3 24 double imag1 3456x4608x3 47775744 uint8
We can investigate the properties of the image with imtool
imtool(imag1)
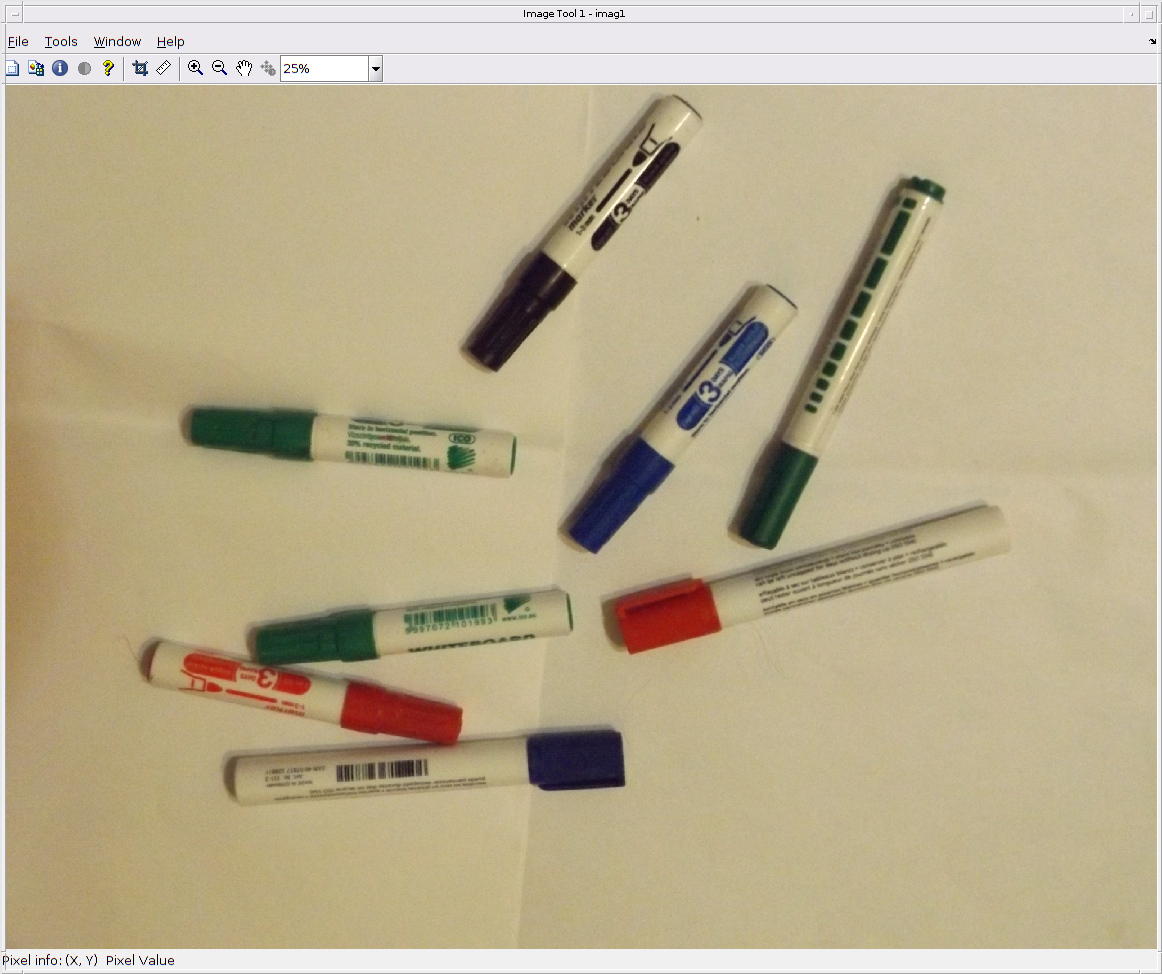
Seratation of colors
redc=imag1(:,:,1);
This contains only the information about the intensity of the red pixels. It's a two dimensional array (a matrix), can be represented as a gray image.
greenc=imag1(:,:,2); bluec=imag1(:,:,3);
Let us plot the different color intensities!
figure subplot(2,2,1) imshow(redc) title('Red') subplot(2,2,2) imshow(greenc) title('Green') subplot(2,2,3) imshow(bluec) title('Blue') subplot(2,2,4) imshow(imag1) title('Original')
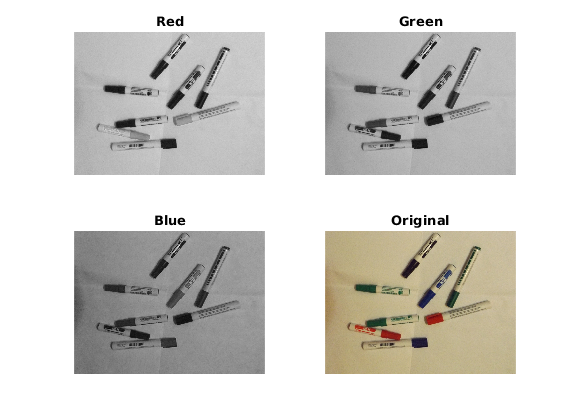
Segmentation
We create a black and white image from the gray image by having a threshold: the pixel whose intensity is higher then the threshold will be white, the rest will be black.
mask_red=im2bw(redc,0.5);
This is a 0-1 matrix, can be represented as logical matrix.
We also use the green color information, because red caps has low intensity of green pixels, while the background has high. This way we can differentiate the background from the caps.
mask_green=~im2bw(greenc,0.25);
Since they are logical we can take the element-wise and operator, tho locate the pixels where the image contains a lot of red but only a few green.
mask2=mask_red & mask_green; subplot(2,2,1) imshow(mask_red) % the background is also white! title('Red') subplot(2,2,2) imshow(mask_green); title('Not green') subplot(2,2,3) imshow(mask2); title('Both masks') subplot(2,2,4) imshow(imag1) title('Original')
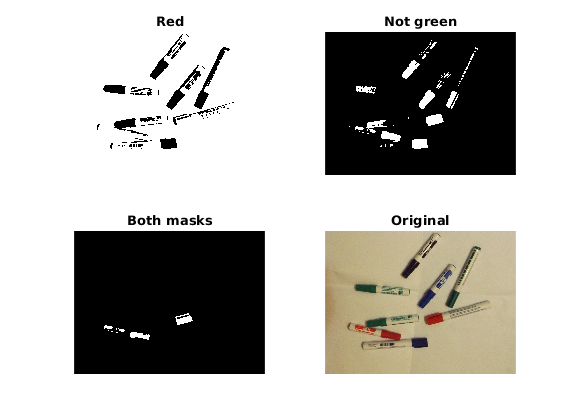
Tiny particles
The function bwareaopen deletes the small particles for example this command deletes the tiny white areas, which are smaller then 30000 pixels.
mask3=bwareaopen(mask2,30000); figure imshow(mask3)
Warning: Image is too big to fit on screen; displaying at 25%
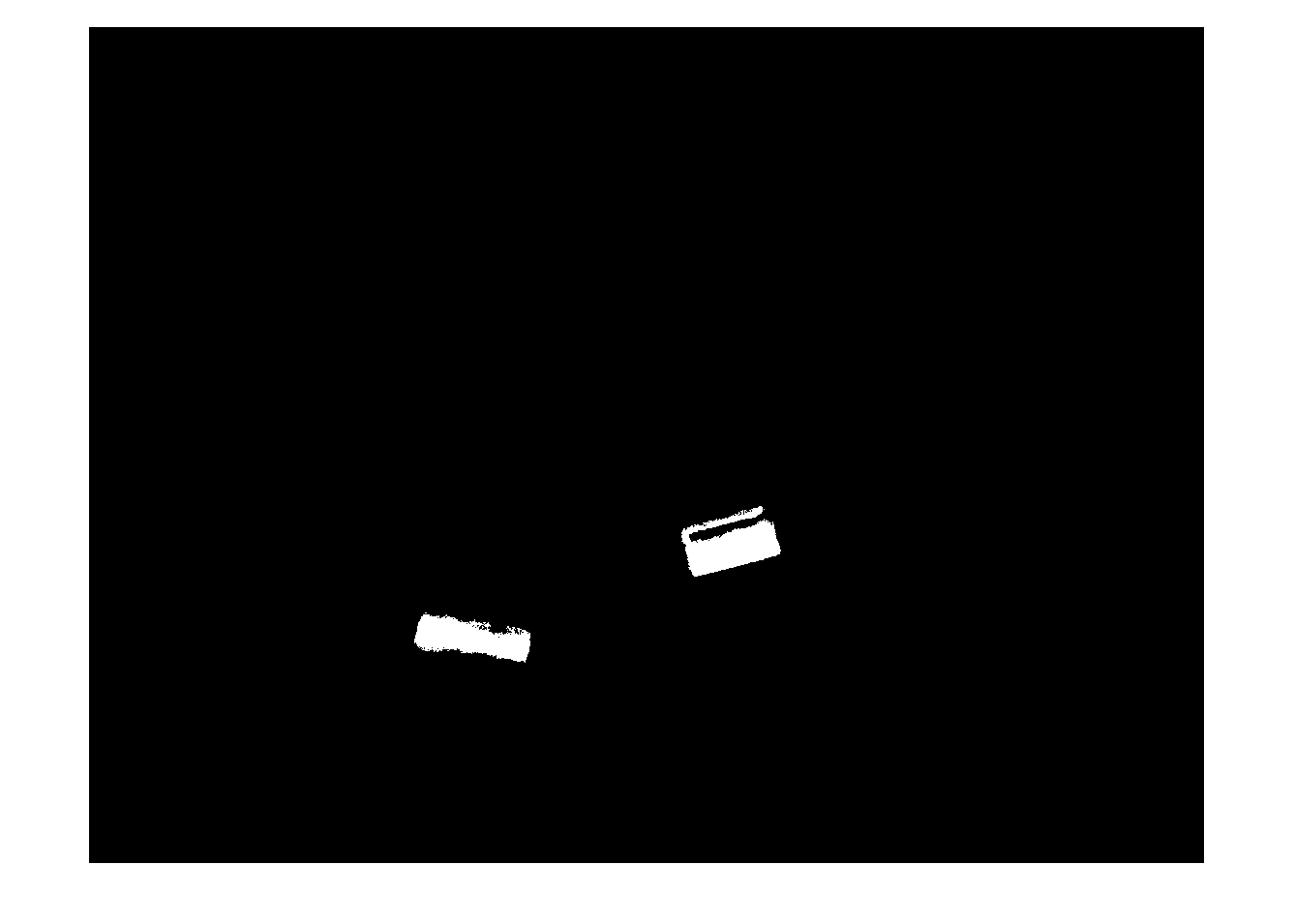
Locating objects
The regionprops function locates the white areas on our image, and gives back a struct which contains the information on these areas location.
caps=regionprops(mask3, 'BoundingBox');
The number of caps found:
size(caps,1)
ans = 2
Plotting the bounding boxes:
imshow(imag1) for i=1:size(caps,1) rectangle('Position',caps(i).BoundingBox,'LineWidth',2,'EdgeColor','g') end
Warning: Image is too big to fit on screen; displaying at 25%
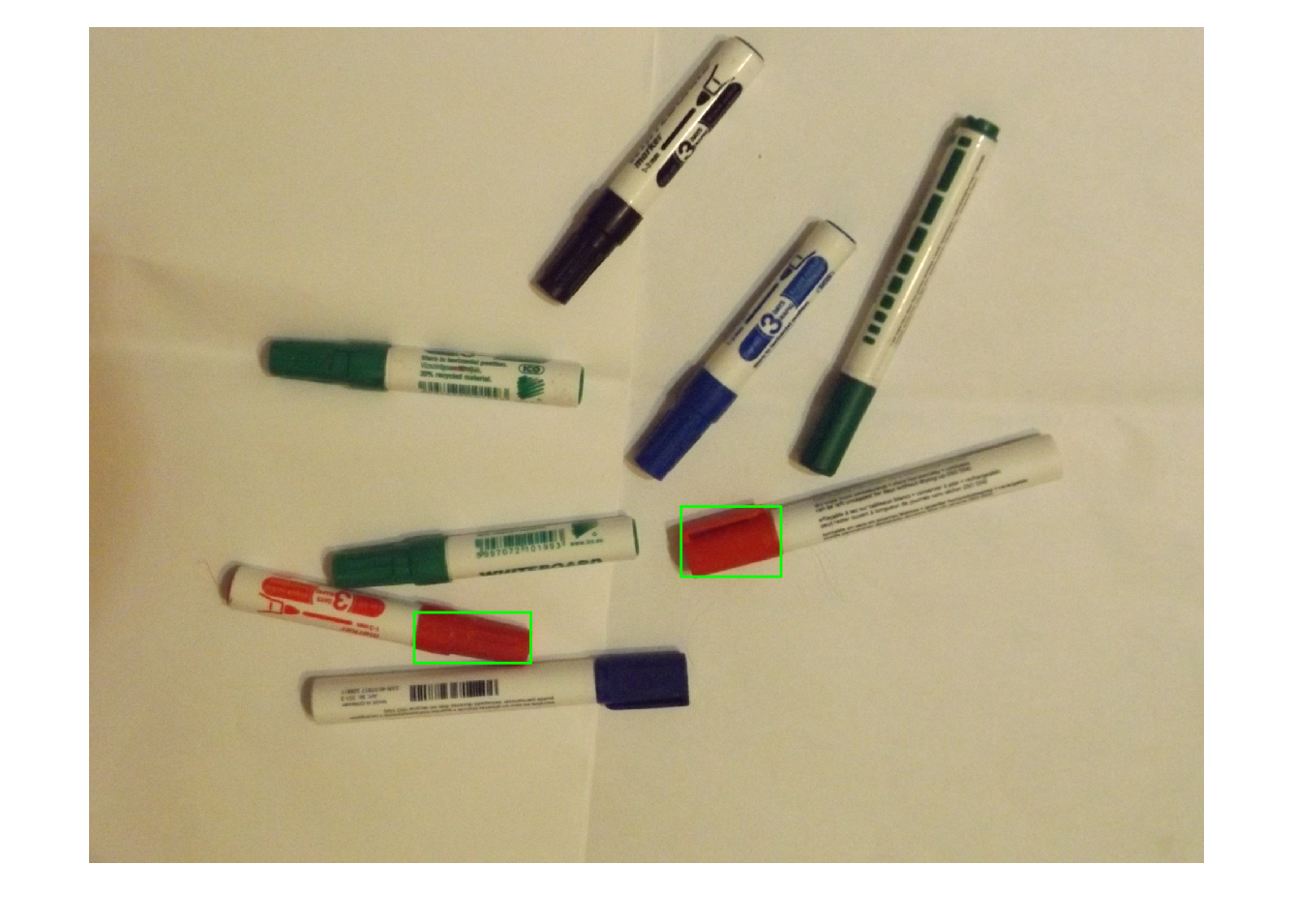
Other image
imag1=imread('050.jpg');
figure
imshow(imag1);
redc=imag1(:,:,1);
greenc=imag1(:,:,2);
bluec=imag1(:,:,3);
mask_red=im2bw(redc,0.5);
mask_green=~im2bw(greenc,0.25);
mask2=mask_red & mask_green;
mask3=bwareaopen(mask2,30000);
Warning: Image is too big to fit on screen; displaying at 25%

caps=regionprops(mask3, 'BoundingBox'); size(caps,1) figure imshow(imag1) for i=1:size(caps,1) rectangle('Position',caps(i).BoundingBox,'LineWidth',2,'EdgeColor','g') end
ans = 2 Warning: Image is too big to fit on screen; displaying at 25%
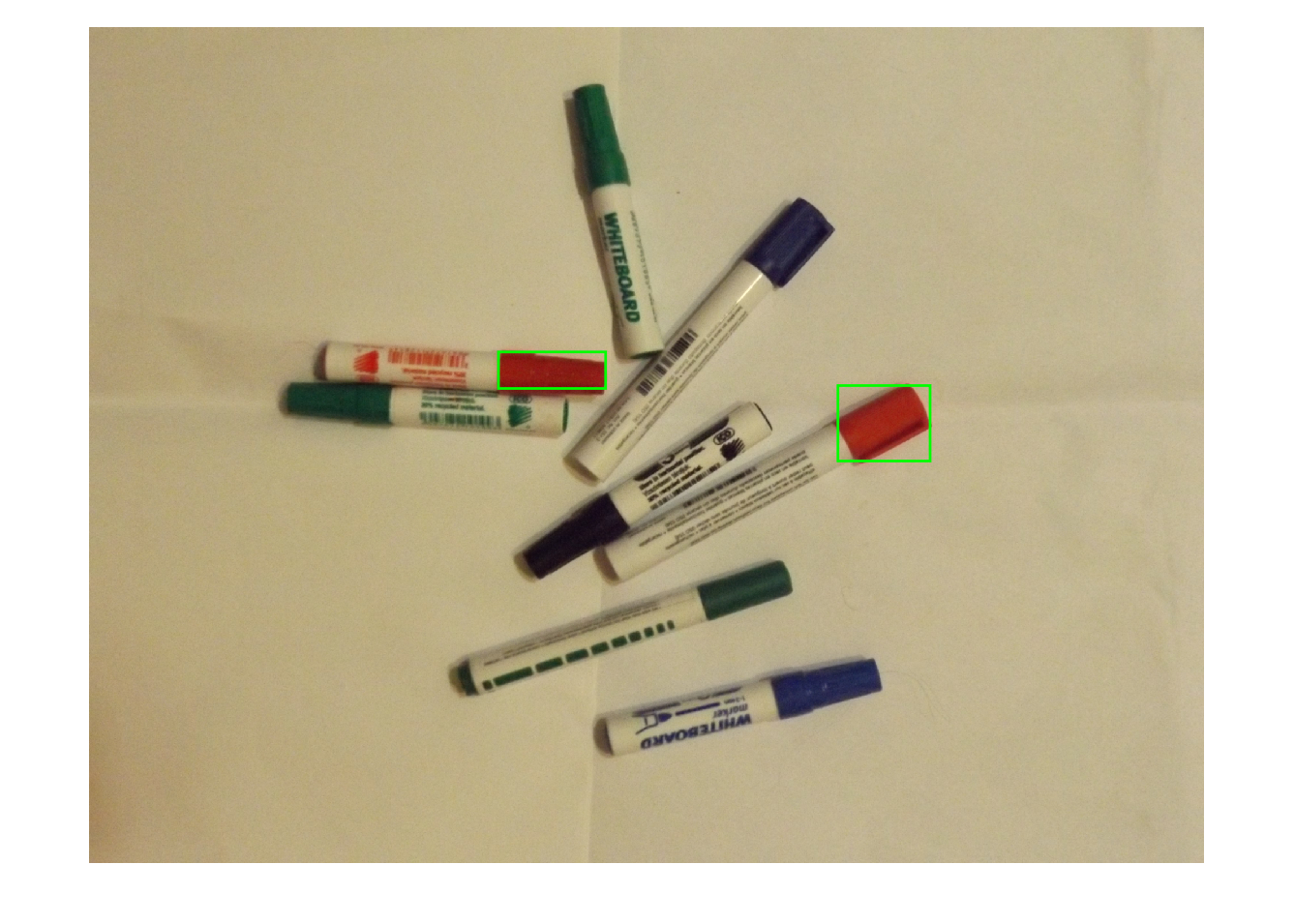
Green whiteboard markers
imag1=imread('043.jpg'); imtool(imag1) % Since the difference between the green and blue caps is small, we have to % use all three colors: redc=imag1(:,:,1); greenc=imag1(:,:,2); bluec=imag1(:,:,3);
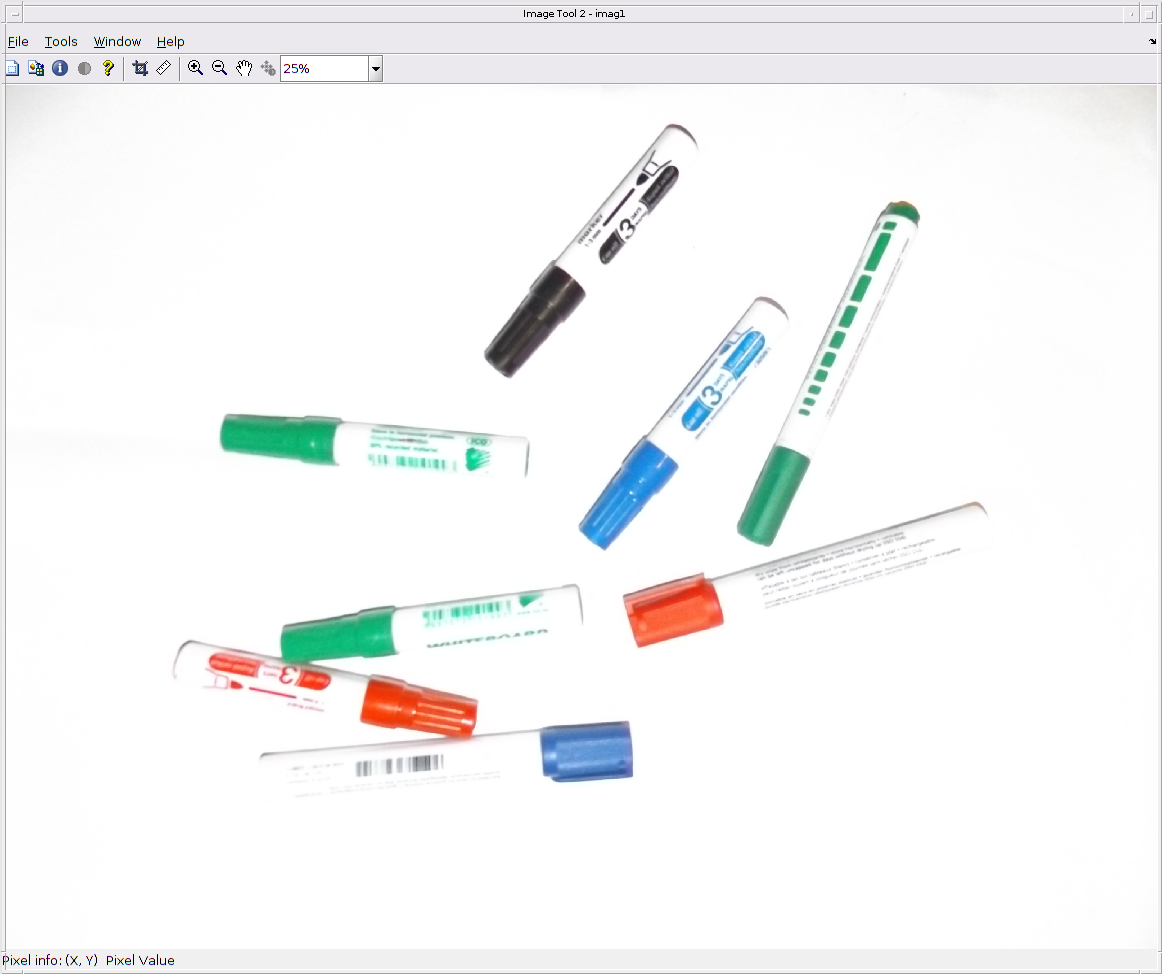
mask_green= im2bw(greenc,0.45); mask_red=~im2bw(redc,0.25); mask_blue= ~im2bw(bluec,0.5); mask2=mask_red & mask_blue & mask_green; imshow(mask2)
Warning: Image is too big to fit on screen; displaying at 25%
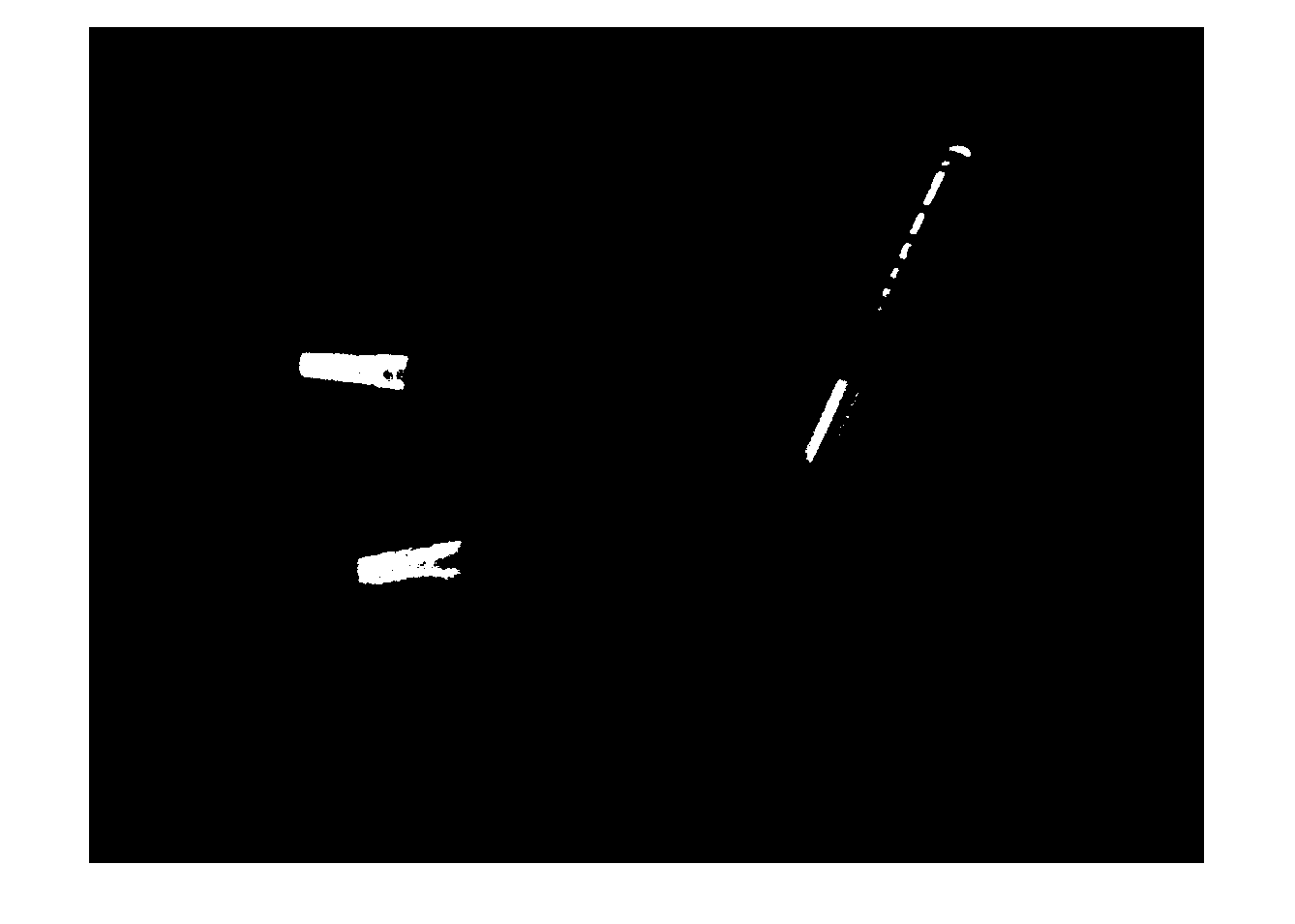
One of the caps is smaller, so we have to decrease the number of pixels in the definition of 'small area'
mask3=bwareaopen(mask2,10000); imshow(mask3)
Warning: Image is too big to fit on screen; displaying at 25%
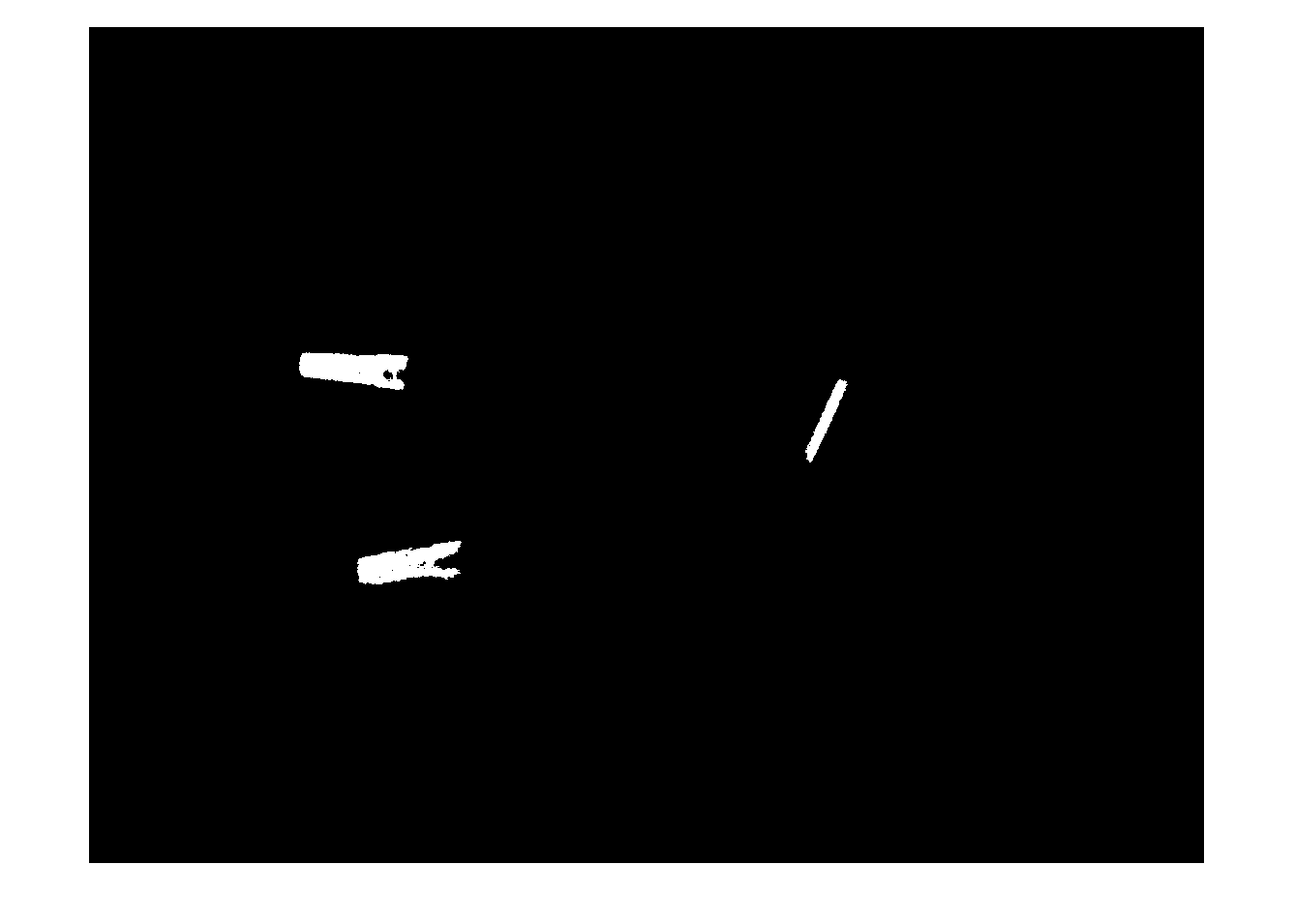
caps=regionprops(mask3, 'BoundingBox'); size(caps,1) imshow(imag1) for i=1:size(caps,1) rectangle('Position',caps(i).BoundingBox,'LineWidth',2,'EdgeColor','r') end
ans = 3 Warning: Image is too big to fit on screen; displaying at 25%
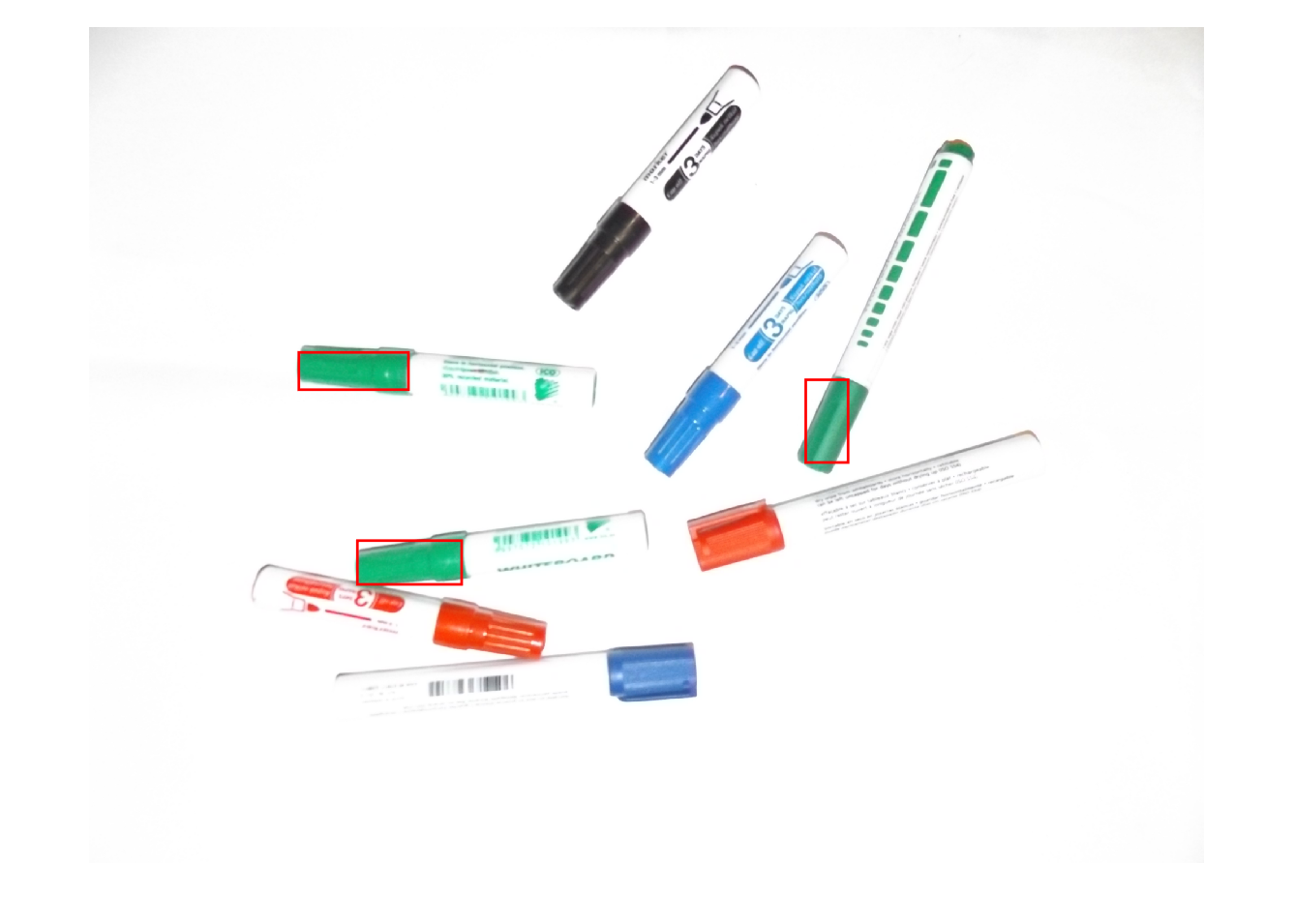
Recommended problems
1: How many pearls are on the picture pearls.jpg?
2: How may blue pearls are in the picture pearls2.jpg?
3: How many birds are on birds.jpg?